Mathematical properties
Numerics.NET provides access to all common mathematical properties of vectors. Because of the cost involved in computing these properties, in particular for large vectors, these properties have been implemented as methods.
General properties
The Length property returns the number of elements in the vector.
The ToArray method returns an array containing the elements of a vector. This method always returns a new array.
The following example illustrates these properties:
var v = Vector.Create(1.0, 2.0, 4.0, 8.0);
var length = v.Length; // 4
var elements = v.ToArray(); // [ 1.0, 2.0, 4.0, 8.0 ]
Vector Norms
The norm of a vector is a measure for the size of the vector. Most common is the Euclidean or two-norm, defined as the square root of the sum of the squares of the elements. The Norm method, without arguments, returns the Euclidean norm. The NormSquared method returns the square of the two-norm, which is the same as the sum of the squares of the elements.
The one-norm of a vector is the sum of the absolute values of its elements. The OneNorm method returns this value.
Other norms can be defined. In general, the p-norm of a vector a with elements ai is defined as
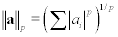
The parameter p can be any real number. If p is negative and one of the elements is zero, then the norm is always zero. Two overloads of the Norm method provide optimized norm calculations. The most commonly used values of p, along with their meaning, are summarized in the table below:
Value of p | Meaning | Method call |
---|---|---|
0 | Number of elements. | |
1 | Sum of absolute values. | |
2 | Euclidean norm. | |
+Infinity | Largest absolute value. | |
-Infinity | Smallest absolute value. |
The following example illustrates the various norm methods:
var v = Vector.Create(1.0, 2.0, 3.0, 4.0, 5.0);
// No arguments: the standard Euclidean norm:
var norm = v.Norm();
// norm -> 7.461...
var oneNorm = v.OneNorm();
var oneNorm2 = v.Norm(1);
// oneNorm -> 15
var maxNorm = v.Norm(Double.PositiveInfinity);
// maxNorm -> 5.0
var minNorm = v.Norm(Double.NegativeInfinity);
// minNorm -> 1.0
// "Zero" norm: the length of the vector
var norm0 = v.Norm(0);
// norm0 -> 5
Extreme values
Methods for finding the smallest and largest elements of a vector, as well as the index of the element with the extreme value. The following table summarizes the methods that can be used to obtain the value or index of an extreme value:
Method (value) | Method (index) | Description |
---|---|---|
Element with largest value | ||
Element with smallest value | ||
Element with largest absolute value | ||
Element with smallest absolute value |